Archive for September 2008
public static void main(String args[])
{
//your code......
}
Next, the JVM runs everything between the curly braces{} of your main method. Every Java application has to have at least one Class, and at least one main method (not one main per class; just one main per application).
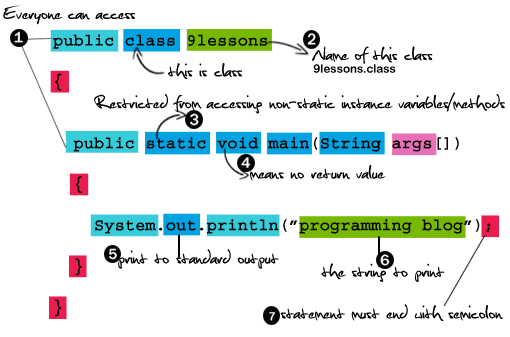
In Java, everything goes in a class. Your'll type your source code file (with a .java extension), then compile it into a new class file (with a .class extension). When your run your program, you're really running a class.
Running a program means telling the Java Virtual Machine (JVM) to "Load the 9lessons class, then start executing its main() method. Keep running till all the code in main is finished.
The main() method is where your program starts running.
9lessons.java
public class 9lessons
{
public static void main(String[] args)
{
System.out.println("Programming Blog");
}
}

step 1: javac 9lessons.java
step 2: java 9lessons
The Big Difference Between public class and Default class
9lessons.java
public class program
{
public static void main(String[] args)
{
System.out.println("Programming Blog");
}
}
Above programe file name is (9lessons.java) and public class name (program) is different

Error : Class program is public, should be declared in a file named program.java
So public class means file name and class name must be same..
Here Default Class(no public)
9lessons.java
class program
{
public static void main(String[] args)
{
System.out.println("Programming Blog");
}
}

After compiling 9lesson.java creates program.class

In the Defalult class no need to give file name and class name same..
step 1: javac 9lessons.java
step 2: java program
If any mistakes please comment me..
Related Articles:
Connecting JSP To Mysql Database Lesson
Web.xml Deployment Descriptor
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Analysis of a Java Class
Google Search Major Data Structures Documentation
Previous Article Link : Google Search Architecture Diagram Overview
Google's data structures are optimized so that a large document collection can be crawled, indexed, and searched with little cost. Although, CPUs and bulk input output rates have improved dramatically over the years, a disk seek still requires about 10 ms to complete. Google is designed to avoid disk seeks whenever possible, and this has had a considerable influence on the design of the data structures.
1. BigFiles
BigFiles are virtual files spanning multiple file systems and are addressable by 64 bit integers. The allocation among multiple file systems is handled automatically. The BigFiles package also handles allocation and deallocation of file descriptors, since the operating systems do not provide enough for our needs. BigFiles also support rudimentary compression options.
2.Repository
The repository contains the full HTML of every web page. Each page is compressed using zlib (see RFC1950). The choice of compression technique is a tradeoff between speed and compression ratio. We chose zlib's speed over a significant improvement in compression offered by bzip. The compression rate of bzip was approximately 4 to 1 on the repository as compared to zlib's 3 to 1 compression. In the repository, the
Repository Data Structure

documents are stored one after the other and are prefixed by docID, length, and URL as can be seen in Figure. The repository requires no other data structures to be used in order to access it. This helps with data consistency and makes development much easier; we can rebuild all the other data structures from only the repository and a file which lists crawler errors.
3. Document Index
The document index keeps information about each document. It is a fixed width ISAM (Index sequential access mode) index, ordered by docID. The information stored in each entry includes the current document status, a pointer into the repository, a document checksum, and various statistics. If the document has been crawled, it also contains a pointer into a variable width file called docinfo which contains its URL and title. Otherwise the pointer points into the URLlist which contains just the URL. This design decision was driven by the desire to have a reasonably compact data structure, and the ability to fetch a record in one disk seek during a search
Additionally, there is a file which is used to convert URLs into docIDs. It is a list of URL checksums with their corresponding docIDs and is sorted by checksum. In order to find the docID of a particular URL, the URL's checksum is computed and a binary search is performed on the checksums file to find its docID. URLs may be converted into docIDs in batch by doing a merge with this file. This is the technique the URLresolver uses to turn URLs into docIDs. This batch mode of update is crucial because otherwise we must perform one seek for every link which assuming one disk would take more than a month for our 322 million link dataset.
4. Lexicon
The lexicon has several different forms. One important change from earlier systems is that the lexicon can fit in memory for a reasonable price. In the current implementation we can keep the lexicon in memory on a machine with 256 MB of main memory. The current lexicon contains 14 million words (though some rare words were not added to the lexicon). It is implemented in two parts -- a list of the words (concatenated together but separated by nulls) and a hash table of pointers. For various functions, the list of words has some auxiliary information which is beyond the scope of this paper to explain fully.
5. Hit Lists
A hit list corresponds to a list of occurrences of a particular word in a particular document including position, font, and capitalization information. Hit lists account for most of the space used in both the forward and the inverted indices. Because of this, it is important to represent them as efficiently as possible. We considered several alternatives for encoding position, font, and capitalization -- simple encoding (a triple of integers), a compact encoding (a hand optimized allocation of bits), and Huffman coding. In the end we chose a hand optimized compact encoding since it required far less space than the simple encoding and far less bit manipulation than Huffman coding. The details of the hits are shown in Figure.
Our compact encoding uses two bytes for every hit. There are two types of hits: fancy hits and plain hits. Fancy hits include hits occurring in a URL, title, anchor text, or meta tag. Plain hits include everything else. A plain hit consists of a capitalization bit, font size, and 12 bits of word position in a document . Font size is represented relative to the rest of the document using three bits (only 7 values are actually used because 111 is the flag that signals a fancy hit). A fancy hit consists of a capitalization bit, the font size set to 7 to indicate it is a fancy hit, 4 bits to encode the type of fancy hit, and 8 bits of position. For anchor hits, the 8 bits of position are split into 4 bits for position in anchor and 4 bits for a hash of the docID the anchor occurs in. This gives us some limited phrase searching as long as there are not that many anchors for a particular word. We expect to update the way that anchor hits are stored to allow for greater resolution in the position and docIDhash fields. We use font size relative to the rest of the document because when searching, you do not want to rank otherwise identical documents differently just because one of the documents is in a larger font.
Forward and Reverse Indexes and the Lexicon

The length of a hit list is stored before the hits themselves. To save space, the length of the hit list is combined with the wordID in the forward index and the docID in the inverted index. This limits it to 8 and 5 bits respectively (there are some tricks which allow 8 bits to be borrowed from the wordID). If the length is longer than would fit in that many bits, an escape code is used in those bits, and the next two bytes contain the actual length.
6. Forward Index
The forward index is actually already partially sorted. It is stored in a number of barrels (we used 64). Each barrel holds a range of wordID's. If a document contains words that fall into a particular barrel, the docID is recorded into the barrel, followed by a list of wordID's with hitlists which correspond to those words. This scheme requires slightly more storage because of duplicated docIDs but the difference is very small for a reasonable number of buckets and saves considerable time and coding complexity in the final indexing phase done by the sorter. Furthermore, instead of storing actual wordID's, we store each wordID as a relative difference from the minimum wordID that falls into the barrel the wordID is in. This way, we can use just 24 bits for the wordID's in the unsorted barrels, leaving 8 bits for the hit list length.
7. Inverted Index
The inverted index consists of the same barrels as the forward index, except that they have been processed by the sorter. For every valid wordID, the lexicon contains a pointer into the barrel that wordID falls into. It points to a doclist of docID's together with their corresponding hit lists. This doclist represents all the occurrences of that word in all documents.
An important issue is in what order the docID's should appear in the doclist. One simple solution is to store them sorted by docID. This allows for quick merging of different doclists for multiple word queries. Another option is to store them sorted by a ranking of the occurrence of the word in each document. This makes answering one word queries trivial and makes it likely that the answers to multiple word queries are near the start. However, merging is much more difficult. Also, this makes development much more difficult in that a change to the ranking function requires a rebuild of the index. We chose a compromise between these options, keeping two sets of inverted barrels -- one set for hit lists which include title or anchor hits and another set for all hit lists. This way, we check the first set of barrels first and if there are not enough matches within those barrels we check the larger ones.
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Google Search Major Data Structures Documentation
In Google, the web crawling (downloading of web pages) is done by several distributed crawlers. There is a URLserver that sends lists of URLs to be fetched to the crawlers. The web pages that are fetched are then sent to the storeserver. The storeserver then compresses and stores the web pages into a repository.Every web page has an associated ID number called a docID which is assigned whenever a new URL is parsed out of a web page. The indexing function is performed by the indexer and the sorter. The indexer performs a number of functions. It reads the repository, uncompresses the documents, and parses them. Each document is converted into a set of word occurrences called hits.
High Level Google Architecture

The hits record the word, position in document, an approximation of font size, and capitalization. The indexer distributes these hits into a set of "barrels", creating a partially sorted forward index. The indexer performs another important function. It parses out all the links in every web page and stores important information about them in an anchors file. This file contains enough information to determine where each link points from and to, and the text of the link.
The URLresolver reads the anchors file and converts relative URLs into absolute URLs and in turn into docIDs. It puts the anchor text into the forward index, associated with the docID that the anchor points to. It also generates a database of links which are pairs of docIDs. The links database is used to compute PageRanks for all the documents.

The sorter takes the barrels, which are sorted by docID, and resorts them by wordID to generate the inverted index. This is done in place so that little temporary space is needed for this operation. The sorter also produces a list of wordIDs and offsets into the inverted index. A program called DumpLexicon takes this list together with the lexicon produced by the indexer and generates a new lexicon to be used by the searcher. The searcher is run by a web server and uses the lexicon built by DumpLexicon together with the inverted index and the PageRanks to answer queries.
Next Article LinkGoogle Search Major Data Structures Documentation
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Google Search Architecture Overview
Tomcat Directory Structure

Login.java
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
public class Login extends HttpServlet{
public void doGet(HttpServletRequest request,
HttpServletResponse response)throws IOException{
PrintWriter out=response.getWriter();
java.util.Date tody=new java.util.Date();
out.println("out put html tags");
}
}
<servlet>Maps internal name to fully-qualified class name.
<servlet-mapping>Maps internal name to public URL name.
web.xml
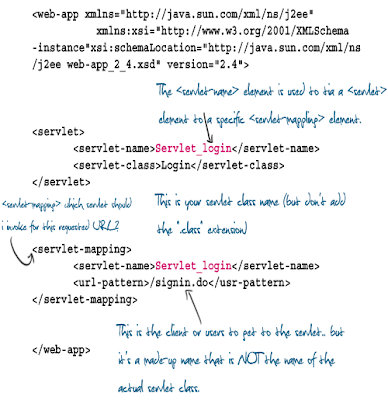
<servlet-mapping> which servlet should i invoke for this requested URL?
<web-app xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee web-app_2_4.xsd" version="2.4">
<servlet>
<servlet-name>Servlet_login</servlet-name>
<servlet-class>Login</servlet-class>
</servlet>
<servlet>
<servlet-name>Servlet_inbox</servlet-name>
<servlet-class>inbox</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>Servlet_login</servlet-name>
<url-pattern>/signin.do</usr-pattern>
</servlet-mapping>
<servlet-mapping>
<servlet-name>Servlet_inbox</servlet-name>
<url-pattern>/view.do</usr-pattern>
</servlet-mapping>
</web-app>
signin.do file mapping to Login.class. So the client or users to get to the servlet.. but it's a made-up name that is NOT the name of the actual servlet class.

Rating: 4.5
Reviewer: Unknown
ItemReviewed: Web.xml Deployment Descriptor
What's in a Database?
a database is a container that holds tables and other SQL structures related to those tables.
An easier way to diagram accout table.
Every one having different hobbies. Remove the hobbiles column and put it in its own table
Add name colum that will let us identify which hobbies belong to which person in the account table.
Linking two tables in a diagram

Connecting Two tables

The problem we're trying to use name field to somehow let use connect the two tables. But what if two people in the accout table have the same name ?

Foreing Key Facts :
A foreign key can have a different name that the primary key it comes form.
The primary key used by a foreign key is also knownas a parent key. the table where the primary key is from is knows as a parent table.
The foreing key can be used to make sure that the rows in on table have corresponding rows in another table.
Foreign key values can be null, even thugh primary key values can't.
Foreign key don't have to be unique - in facts, they ofter aren't
CREATE TABLE accout(
user_id INT NOT NULL AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(20),
email VARCHAR(30),
birthday DATE,
gender VARCHAR(10),
city VARCHAR(30));

CREATE TABLE hobbies(
hob_id INT NOT NULL AUTO_INCREMENT PRIMARY KEY,hobby VARCHAR(100) NOT NULL,
user_id INT NOT NULL,
CONSTRAINT account_user_id_fK
FOREIGN KEY(user_id)
REFERENCES accout(user_id));

Rating: 4.5
Reviewer: Unknown
ItemReviewed: What is FOREIGN KEY? Easy Lesson

Login.html

Code :
<form action="login.jsp" method="post">
User name :<input type="text" name="usr" />
password :<input type="password" name="pwd" />
<input type="submit" />
Reg.html

code:
Email :<input type="text" name="email" />
First name :<input type="text" name="fname" />
Last name :<input type="text" name="lname" />
User name :<input type="text" name="userid" />
password :<input type="password" name="pwd" />
<input type="submit" />
</form>
Mysql Create Database Test:
Mysql no doubt about it best open source database http://mysql.com/

Create Table Users:

login.jsp
<%@ page import ="java.sql.*" %>
<%@ page import ="javax.sql.*" %>
<%
String userid=request.getParameter("user");
session.putValue("userid",userid);
String pwd=request.getParameter("pwd");
Class.forName("com.mysql.jdbc.Driver");
java.sql.Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/
test","root","root");
Statement st= con.createStatement();
ResultSet rs=st.executeQuery("select * from users where user_id='"+userid+"'");
if(rs.next())
{
if(rs.getString(2).equals(pwd))
{
out.println("welcome"+userid);
}
else
{
out.println("Invalid password try again");
}
}
else
%>
reg.jsp
<%@ page import ="java.sql.*" %>
<%@ page import ="javax.sql.*" %>
<%
String user=request.getParameter("userid");
session.putValue("userid",user);
String pwd=request.getParameter("pwd");
String fname=request.getParameter("fname");
String lname=request.getParameter("lname");
String email=request.getParameter("email");
Class.forName("com.mysql.jdbc.Driver");
java.sql.Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/test",
"root","root");
Statement st= con.createStatement();
ResultSet rs;
int i=st.executeUpdate("insert into users values ('"+user+"','"+pwd+"','"+fname+"',
'"+lname+"','"+email+"')");
%>
welcome.jsp
<%@ page import ="java.sql.*" %>
<%@ page import ="javax.sql.*" %>
<%
String user=session.getValue("userid").toString();
%>
Registration is Successfull. Welcome to <%=user %>
Tomcat Directory Structure
Tomcat open source web server you can download from this link http://tomcat.apache.org/

Run Your Project

Rating: 4.5
Reviewer: Unknown
ItemReviewed: Connecting JSP To Mysql Database Lesson
Recently my friend Sreekanth published one website about his company. But Google search database doesn't know about his website. So how to add site URL in Google Search Database.
Goto Google Webmaster Tools Website
Google Verify Your Site URL
Copy and Paste in your site index/home page
Paste this after title tag
After saving the site home/index page click verify buttom.
Add Sitemap every website having Site Structure in XML formate many sitemap generators in internet http://www.xml-sitemaps.com.
But Blogger/Wordpress having defalut sitemape files like atom.xml.
Rating: 4.5
Reviewer: Unknown
ItemReviewed: Add Your Site/Blog URL in Google Search Database.
body {
background-color: #FFFFFF;
font-family: Verdana, Arial;
font-size:20px 0;
color:#5A5A54;
}
#navbar-iframe{
display:none;
height:0px;
visibility:hidden;
}
Finally save the template
Rating: 4.5
Reviewer: Unknown
ItemReviewed: How To Disable Blogger Top Nav Bar
Step 1: Open blogger dashboard
Step 2: Go to layout page select edit html
Step 3: Page down revert Xml template to classic html format
Step 4: Before edit take backup your original template code
If you have any queries just comment.
next lesson how to disable blogger defalt top Navabar
Rating: 4.5
Reviewer: Unknown
ItemReviewed: How to Customize Blogger Template
int *a;
The statement written above means that a is a special variable(i.e. pointer) that will store an address of another variable which is integer.
For eg.
int *a;
int b=10;
a=&b;
then it will be like this-

Here 3465 is address for variable a and 1217 is address for variable b.
NOTE:All variables store integers bcoz address are always integers....
thus if we write
char *a;
It will not mean that a will store character it means that it will store the address of some variable whose datatype is char.
Rating: 4.5
Reviewer: Unknown
ItemReviewed: C Pointers Lesson